
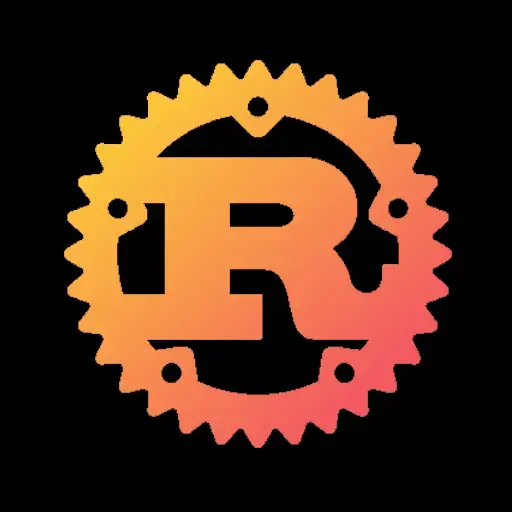
1·
11 months agoApparently there’s an effort underway. I don’t have any more context than this:
https://news.ycombinator.com/item?id=38020117
I will say that I actually like the flat namespace, but don’t have a strong opinion
Apparently there’s an effort underway. I don’t have any more context than this:
https://news.ycombinator.com/item?id=38020117
I will say that I actually like the flat namespace, but don’t have a strong opinion
For a direct replacement, you might want to consider enums, for something like
enum Strategy { Foo, Bar, }
That’s going to be a lot more ergonomic than shuffling trait objects around, you can do stuff like:
fn execute(strategy: Strategy) { match strategy { Strategy::Foo => { ... } Strategy::Bar => { ... } }
If you have known set of strategy that isn’t extensible, enums are good. If you want the ability for third party code to add new strategies, the boxed trait object approach works. Consider also the simplest approach of just having functions like this:
fn execute_foo() { ... } fn execute_bar() { ... }
Sometimes, Rust encourages not trying to be too clever, like having
get
vsget_mut
and not trying to abstract over the mutability.